Flutter의 화면에서 왼쪽배치를 할 것인지, 가운데에 배치할 것이 아니면 위에 아니면 밑에 배치할 것인지 정하게 하는 방법 이 있다
Column일 경우와 Row일 경우에 배치하는 법을 알아보도록 하겠다
1. 기본코드
main.dart
import 'package:flutter/material.dart';
import 'package:flutter_practice/pages/practice_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
home: const PracticePage(),
);
}
}
practice_page.dart
import 'package:flutter/material.dart';
class PracticePage extends StatelessWidget {
const PracticePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
leading: Icon(Icons.car_crash),
title: Text("Appbar"),
actions: [
Icon(Icons.search),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
child: Column(
children: [
Text("HelloWord", style: TextStyle(fontSize: 30)),
Text("HelloWord", style: TextStyle(fontSize: 30)),
],
),
),
);
}
}
일단 이렇게 코드를 작성해보도록 한다.
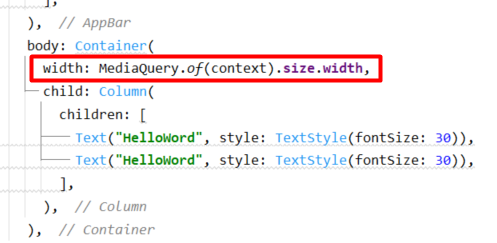
이 부분은 화면의 전체사이즈를 잡아주는 역할을 한다
그 이유는 HelloWord라는 문자열이 짧기 때문에, 정렬예제를 보여줄 수 없어서 넣어줬다.
에뮬레이터로 나중에 설명하도록 한다.
이대로 실행을 해보면 아래와 같이 나온다
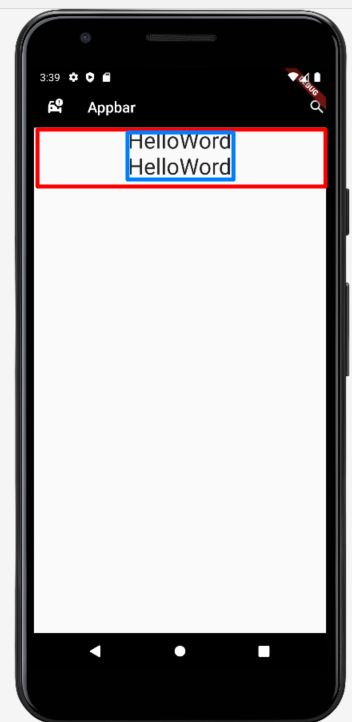
실행을 해보면 HelloWord 두 개가 배치가 되면서
빨간색 네모 부분이 Container고 width: MediaQuery로 넓이를 잡아준 것이라고 보면 된다.
만약 width: MediaQuery가 없다면 왼쪽으로 배치가 되었을 것이다.
기본적으로 가운데 정렬인 듯하다
2. CrossAxisAlignment
CrossAxisAlignment을 사용해 보겠다.
2-1. CrossAxisAlignment.start
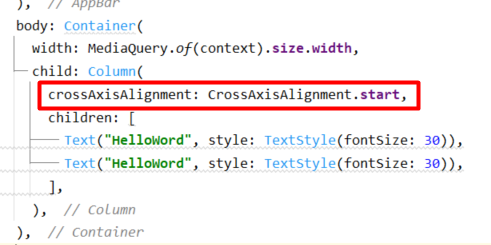
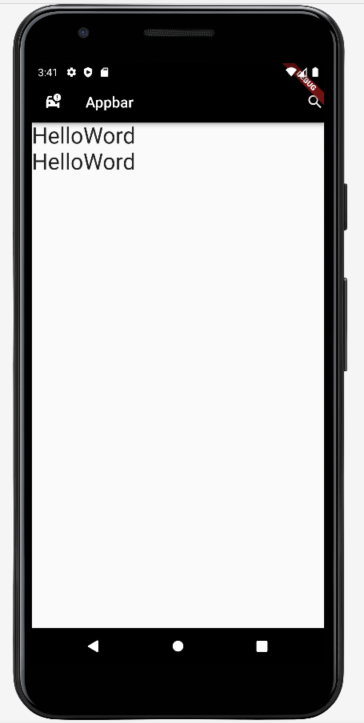
start는 왼쪽으로 정렬이 되었다
2-2. CrossAxisAlignment.center
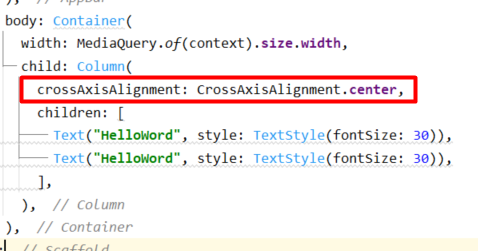
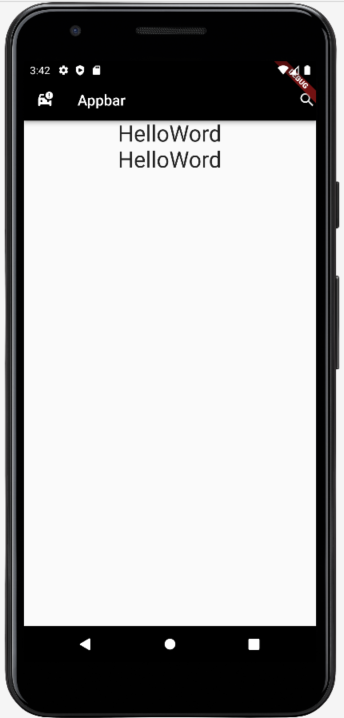
가운데 정렬
2-1. CrossAxisAlignment.end
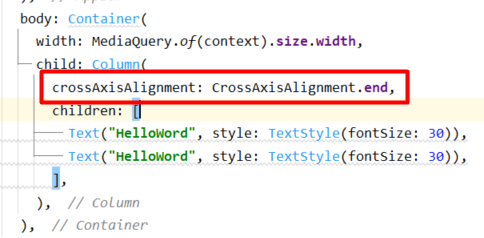

오른쪽 정렬이 되었다
3. MainAxisAlignment
CrossAxisAlignment 말고 MainAxisAlignment를 사용해 보도록 한다
3-1. MainAxisAlignment.start
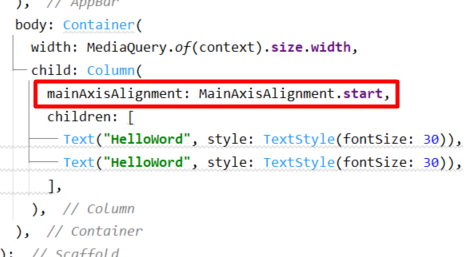

아무것도 안 적은 것과 변화가 없다. 왜일까? 그다음을 보도록 해본다
3-1. MainAxisAlignment.center
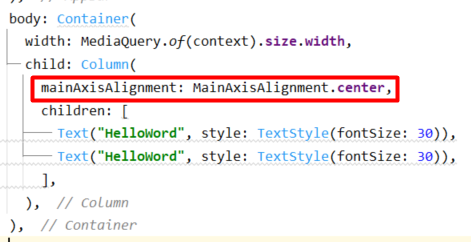
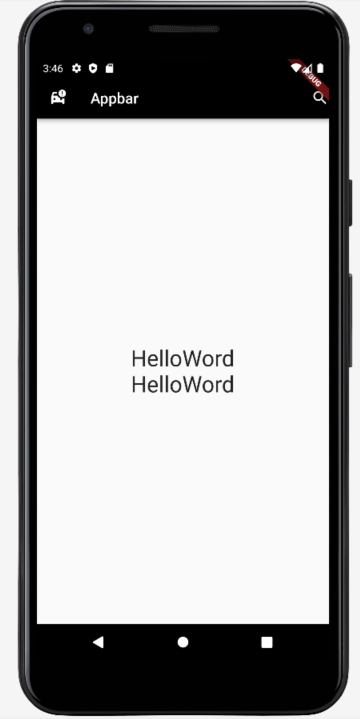
새로 높이에서 한 가운데에 정렬이 되었다.
그렇다 start는 새로 높이에서 맨 위를 뜻한다
3-1. MainAxisAlignment.end
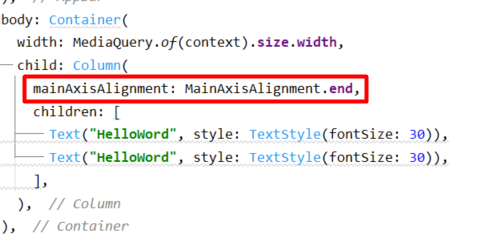
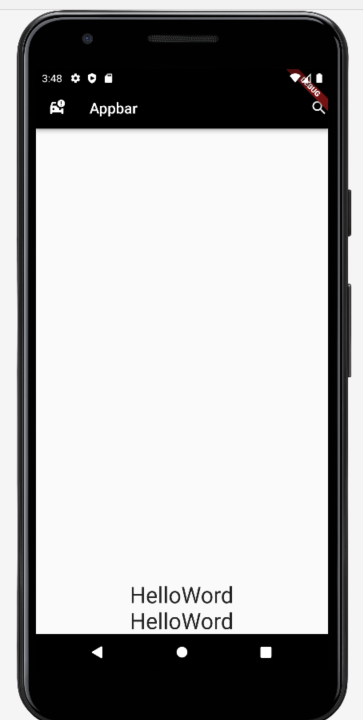
예상대로 end를 넣어주면 맨 밑에 배치가 된다
4. Column이 아니라 Row일 때는 어떨까?
4-1 기본코드
import 'package:flutter/material.dart';
class PracticePage extends StatelessWidget {
const PracticePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
leading: Icon(Icons.car_crash),
title: Text("Appbar"),
actions: [
Icon(Icons.search),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
child: Row(
children: [
Text("HelloWord", style: TextStyle(fontSize: 30)),
Text("HelloWord", style: TextStyle(fontSize: 30)),
],
),
),
);
}
}
코드를 바꿔 보았다.
이번엔 height를 넣어주고 새로 사이즈를 에뮬레이터크기대로 잡아주었다.
그리고 Column부분을 Row로 바꿔보았다
이대로 실행해본다

새로 높이를 주었더니 새로 가운데에 가로 왼쪽정렬이 되어 있다. 이게 기본정렬인 것 같다
4-2. CrossAxisAlignment
4-2-1. CrossAxisAlignment.start
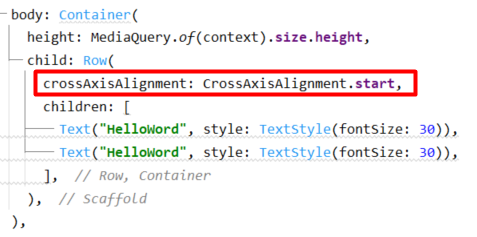
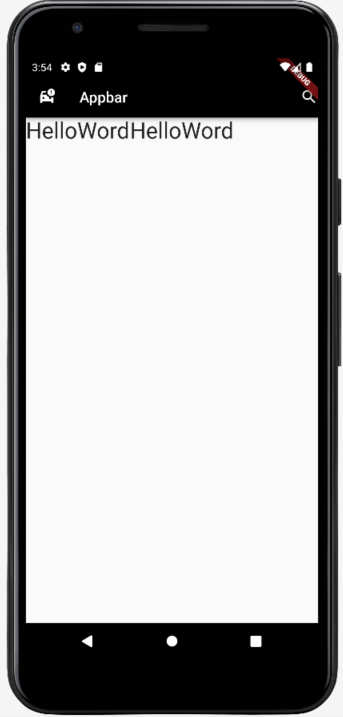
CrossAxisAlignment.start를 주었더니, 위로 배치가 되었다.
Column일 때는 CrossAxisAlignment.start 주면 왼쪽으로 배치가 되는데, Row일 때는 위로 배치가 되었다
반대로 움직인다는 것을 알 수 있다
4-2-2. CrossAxisAlignment.center
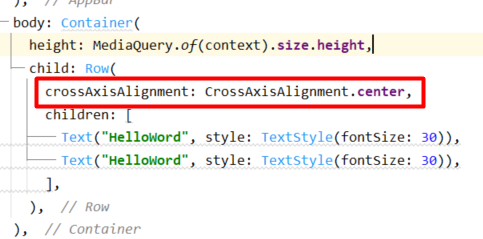

4-2-3. CrossAxisAlignment.end


4-3. MainAxisAlignment
MainAxisAlignment도 한번 실험해 보겠다
CrossAxisAlignment를 지우고 MainAxisAlignment로 사용해 보자
4-3-1. MainAxisAlignment.start
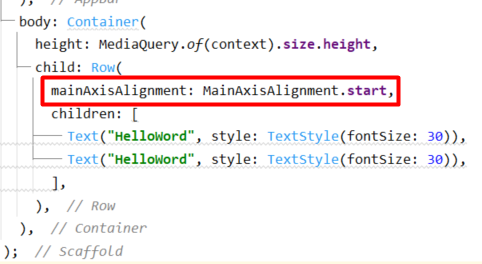

MainAxisAlignment.start를 사용해 보았더니 변화가 없는 것 같지만, 기본이 왼쪽으로 정렬되어 있기 때문에 변화가 없어 보이는 것뿐이다
4-3-2. MainAxisAlignment.center
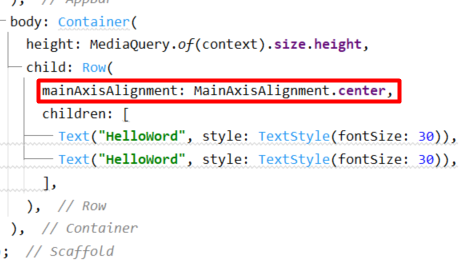
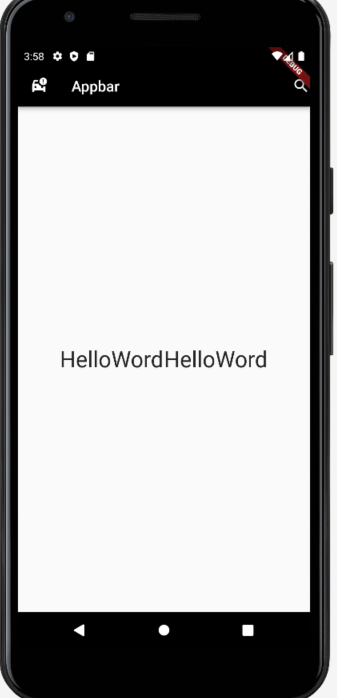
4-3-3. MainAxisAlignment.end
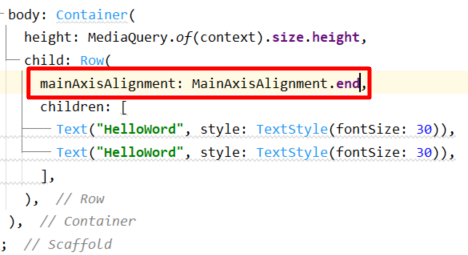
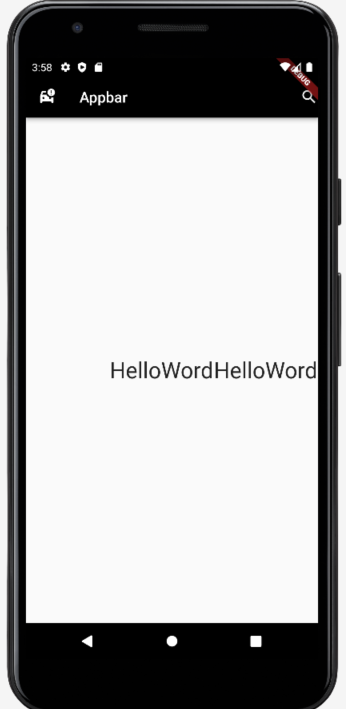
오른쪽 정렬이 되었다.
5. 정리
5-1. Column일 때
5-1-1. CrossAxisAlignment
- CrossAxisAlignment.start: 가로기준 왼쪽 배치
- CrossAxisAlignment.center: 가로기준 중간 배치
- CrossAxisAlignment.end: 가로기준 오른쪽 배치
5-1-2. MainAxisAlignment
- MainAxisAlignment.start: 새로 기준 위에 배치
- MainAxisAlignment.center: 새로기준 중간 배치
- MainAxisAlignment.end: 새로기준 밑에 배치
5-2. Row일 때
5-2-1. CrossAxisAlignment
- CrossAxisAlignment.start: 새로 기준 위에 배치
- CrossAxisAlignment.center: 새로 기준 중간 배치
- CrossAxisAlignment.end: 새로기준 밑에 배치
5-2-2. MainAxisAlignment
- MainAxisAlignment.start: 가로기준 왼쪽 배치
- MainAxisAlignment.center: 가로기준 중간 배치
- MainAxisAlignment.end: 가로기준 오른쪽 배치
조금 복잡하긴 하지만, Column일 때만 외워두고 Row일 때는 반대로 생각하면 될 것 같다
'Web Programming > Flutter&Dart' 카테고리의 다른 글
Flutter 이미지 화면 비율대로 크기 설정 (AspectRatio) (0) | 2023.02.14 |
---|---|
Flutter Image 넣기 (0) | 2023.02.13 |
Flutter의 Padding EdgeInsets (all, only, symmetric차이점) (0) | 2023.02.09 |
ListView와 SingleChildScrollView (새로 스크롤, 가로 스크롤) (1) | 2023.02.08 |
가로, 새로로 배치 하기 (Column과 Row) (0) | 2023.02.07 |
Flutter의 화면에서 왼쪽배치를 할 것인지, 가운데에 배치할 것이 아니면 위에 아니면 밑에 배치할 것인지 정하게 하는 방법 이 있다
Column일 경우와 Row일 경우에 배치하는 법을 알아보도록 하겠다
1. 기본코드
main.dart
import 'package:flutter/material.dart';
import 'package:flutter_practice/pages/practice_page.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
home: const PracticePage(),
);
}
}
practice_page.dart
import 'package:flutter/material.dart';
class PracticePage extends StatelessWidget {
const PracticePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
leading: Icon(Icons.car_crash),
title: Text("Appbar"),
actions: [
Icon(Icons.search),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
child: Column(
children: [
Text("HelloWord", style: TextStyle(fontSize: 30)),
Text("HelloWord", style: TextStyle(fontSize: 30)),
],
),
),
);
}
}
일단 이렇게 코드를 작성해보도록 한다.
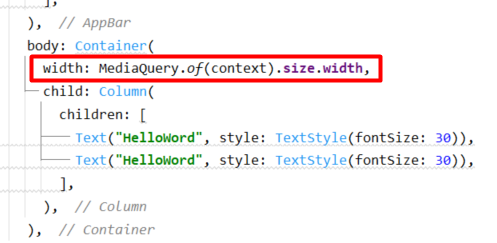
이 부분은 화면의 전체사이즈를 잡아주는 역할을 한다
그 이유는 HelloWord라는 문자열이 짧기 때문에, 정렬예제를 보여줄 수 없어서 넣어줬다.
에뮬레이터로 나중에 설명하도록 한다.
이대로 실행을 해보면 아래와 같이 나온다
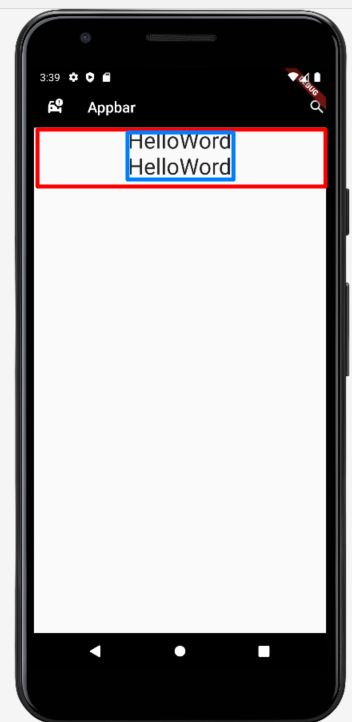
실행을 해보면 HelloWord 두 개가 배치가 되면서
빨간색 네모 부분이 Container고 width: MediaQuery로 넓이를 잡아준 것이라고 보면 된다.
만약 width: MediaQuery가 없다면 왼쪽으로 배치가 되었을 것이다.
기본적으로 가운데 정렬인 듯하다
2. CrossAxisAlignment
CrossAxisAlignment을 사용해 보겠다.
2-1. CrossAxisAlignment.start
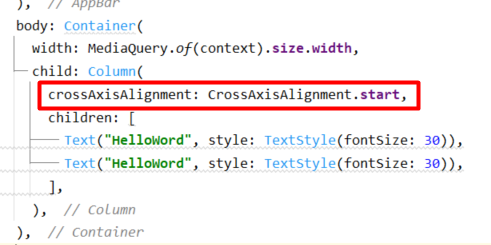
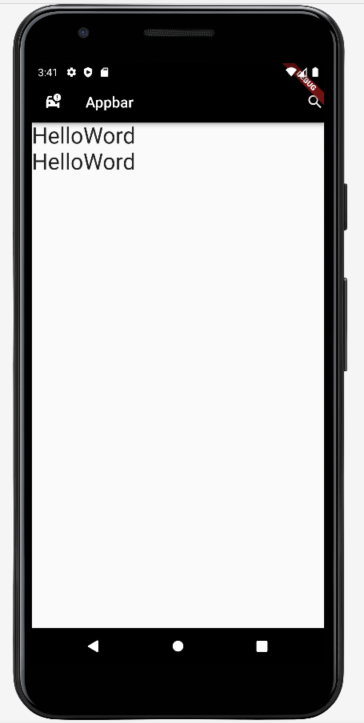
start는 왼쪽으로 정렬이 되었다
2-2. CrossAxisAlignment.center
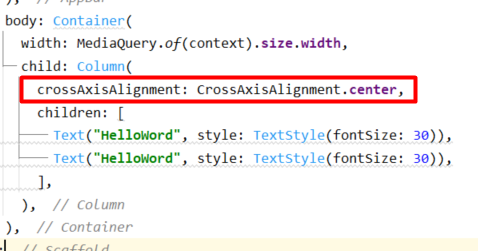
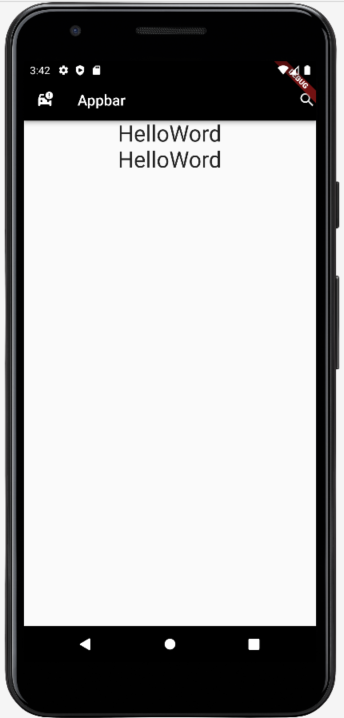
가운데 정렬
2-1. CrossAxisAlignment.end
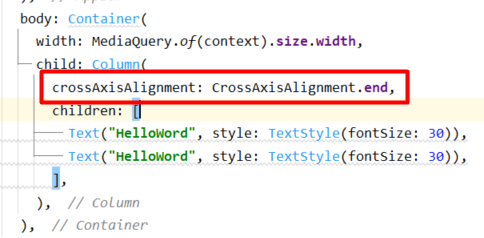

오른쪽 정렬이 되었다
3. MainAxisAlignment
CrossAxisAlignment 말고 MainAxisAlignment를 사용해 보도록 한다
3-1. MainAxisAlignment.start
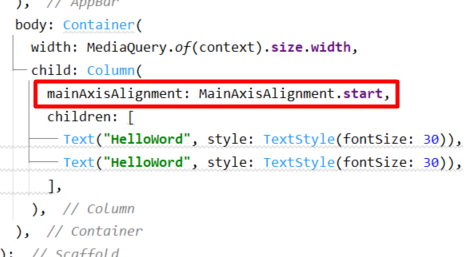

아무것도 안 적은 것과 변화가 없다. 왜일까? 그다음을 보도록 해본다
3-1. MainAxisAlignment.center
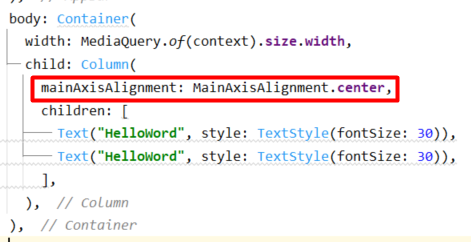
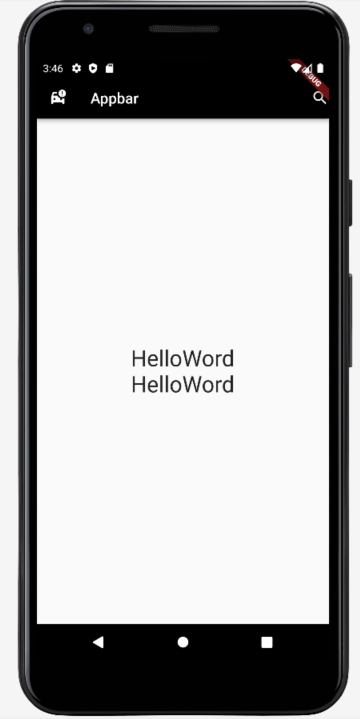
새로 높이에서 한 가운데에 정렬이 되었다.
그렇다 start는 새로 높이에서 맨 위를 뜻한다
3-1. MainAxisAlignment.end
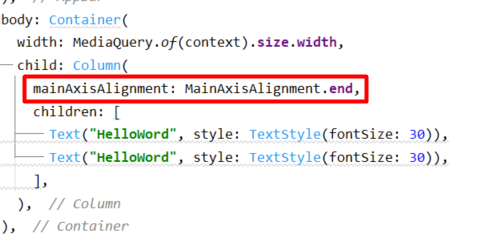
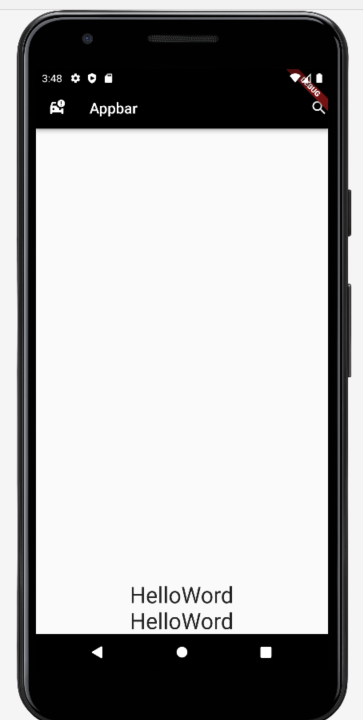
예상대로 end를 넣어주면 맨 밑에 배치가 된다
4. Column이 아니라 Row일 때는 어떨까?
4-1 기본코드
import 'package:flutter/material.dart';
class PracticePage extends StatelessWidget {
const PracticePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.black,
leading: Icon(Icons.car_crash),
title: Text("Appbar"),
actions: [
Icon(Icons.search),
],
),
body: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
child: Row(
children: [
Text("HelloWord", style: TextStyle(fontSize: 30)),
Text("HelloWord", style: TextStyle(fontSize: 30)),
],
),
),
);
}
}
코드를 바꿔 보았다.
이번엔 height를 넣어주고 새로 사이즈를 에뮬레이터크기대로 잡아주었다.
그리고 Column부분을 Row로 바꿔보았다
이대로 실행해본다

새로 높이를 주었더니 새로 가운데에 가로 왼쪽정렬이 되어 있다. 이게 기본정렬인 것 같다
4-2. CrossAxisAlignment
4-2-1. CrossAxisAlignment.start
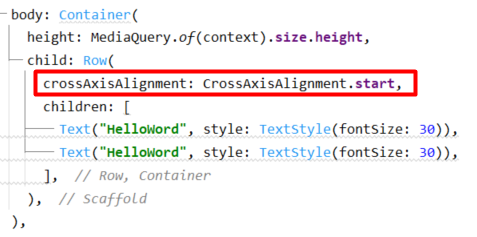
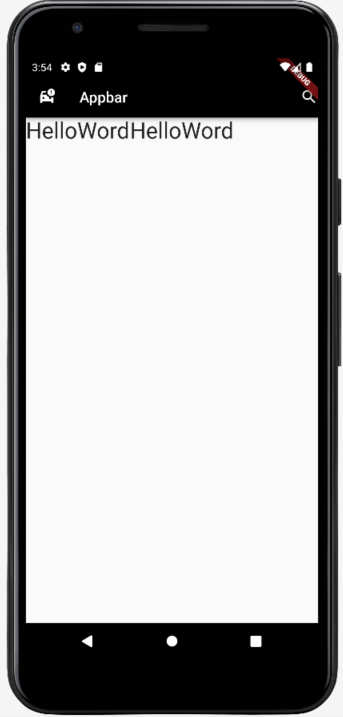
CrossAxisAlignment.start를 주었더니, 위로 배치가 되었다.
Column일 때는 CrossAxisAlignment.start 주면 왼쪽으로 배치가 되는데, Row일 때는 위로 배치가 되었다
반대로 움직인다는 것을 알 수 있다
4-2-2. CrossAxisAlignment.center
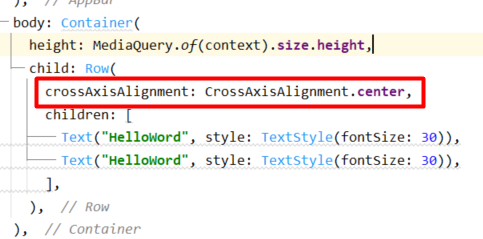

4-2-3. CrossAxisAlignment.end


4-3. MainAxisAlignment
MainAxisAlignment도 한번 실험해 보겠다
CrossAxisAlignment를 지우고 MainAxisAlignment로 사용해 보자
4-3-1. MainAxisAlignment.start
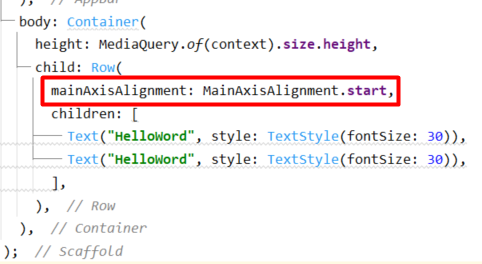

MainAxisAlignment.start를 사용해 보았더니 변화가 없는 것 같지만, 기본이 왼쪽으로 정렬되어 있기 때문에 변화가 없어 보이는 것뿐이다
4-3-2. MainAxisAlignment.center
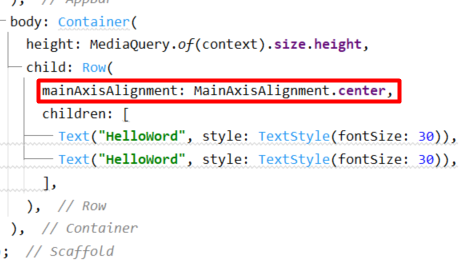
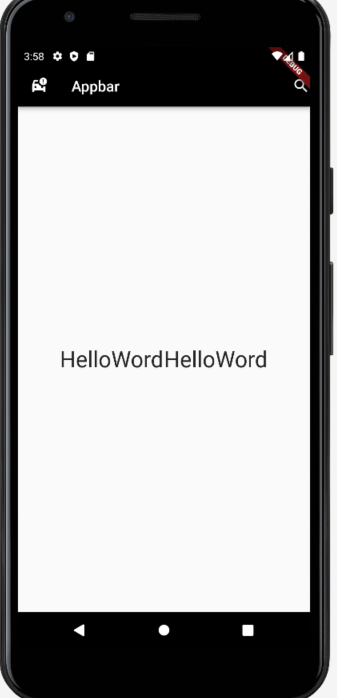
4-3-3. MainAxisAlignment.end
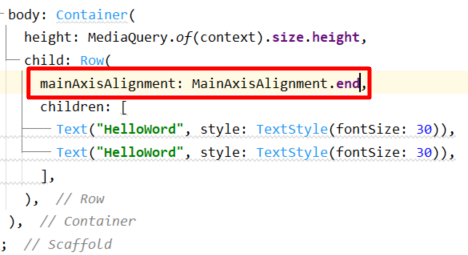
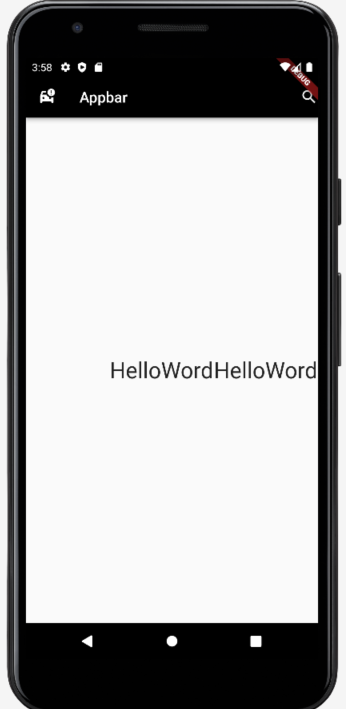
오른쪽 정렬이 되었다.
5. 정리
5-1. Column일 때
5-1-1. CrossAxisAlignment
- CrossAxisAlignment.start: 가로기준 왼쪽 배치
- CrossAxisAlignment.center: 가로기준 중간 배치
- CrossAxisAlignment.end: 가로기준 오른쪽 배치
5-1-2. MainAxisAlignment
- MainAxisAlignment.start: 새로 기준 위에 배치
- MainAxisAlignment.center: 새로기준 중간 배치
- MainAxisAlignment.end: 새로기준 밑에 배치
5-2. Row일 때
5-2-1. CrossAxisAlignment
- CrossAxisAlignment.start: 새로 기준 위에 배치
- CrossAxisAlignment.center: 새로 기준 중간 배치
- CrossAxisAlignment.end: 새로기준 밑에 배치
5-2-2. MainAxisAlignment
- MainAxisAlignment.start: 가로기준 왼쪽 배치
- MainAxisAlignment.center: 가로기준 중간 배치
- MainAxisAlignment.end: 가로기준 오른쪽 배치
조금 복잡하긴 하지만, Column일 때만 외워두고 Row일 때는 반대로 생각하면 될 것 같다
'Web Programming > Flutter&Dart' 카테고리의 다른 글
Flutter 이미지 화면 비율대로 크기 설정 (AspectRatio) (0) | 2023.02.14 |
---|---|
Flutter Image 넣기 (0) | 2023.02.13 |
Flutter의 Padding EdgeInsets (all, only, symmetric차이점) (0) | 2023.02.09 |
ListView와 SingleChildScrollView (새로 스크롤, 가로 스크롤) (1) | 2023.02.08 |
가로, 새로로 배치 하기 (Column과 Row) (0) | 2023.02.07 |